里氏替换原则
里氏替换原则
所有引用基类的地方必须能透明地使用其子类的对象,也就是说子类对象可以替换其父类对象,而程序执行效果不变。
解读: 在继承体系中,子类中可以增加自己特有的方法,也可以实现父类的抽象方法,但是不能重写父类的非抽象方法,否则该继承关系就不是一个正确的继承关系。
类图对比
未实践里氏替换原则:
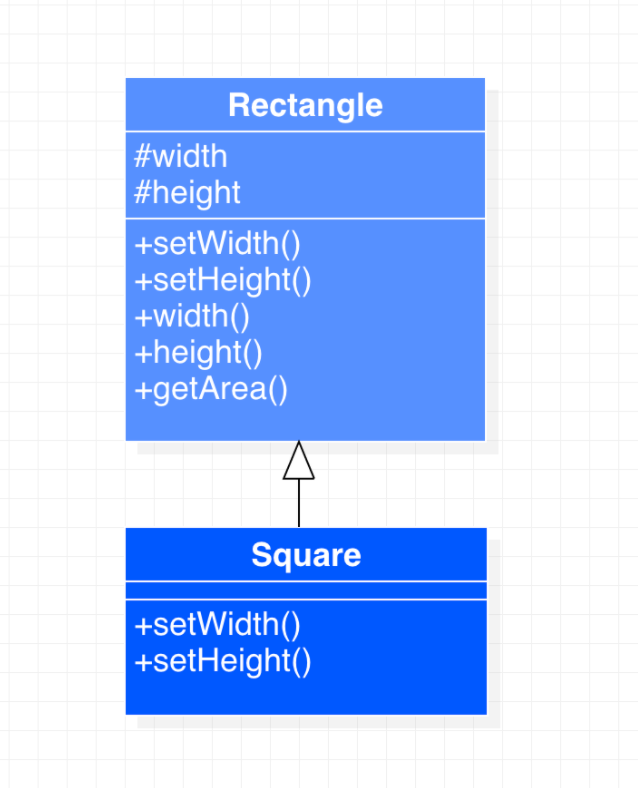
//================== Rectangle.h ==================
@interface Rectangle : NSObject
{
@protected double _width;
@protected double _height;
}
//设置宽高
- (void)setWidth:(double)width;
- (void)setHeight:(double)height;
//获取宽高
- (double)width;
- (double)height;
//获取面积
- (double)getArea;
@end
//================== Rectangle.m ==================
@implementation Rectangle
- (void)setWidth:(double)width{
_width = width;
}
- (void)setHeight:(double)height{
_height = height;
}
- (double)width{
return _width;
}
- (double)height{
return _height;
}
- (double)getArea{
return _width * _height;
}
@end
//================== Square.h ==================
@interface Square : Rectangle
@end
//================== Square.m ==================
@implementation Square
- (void)setWidth:(double)width{
_width = width;
_height = width;
}
- (void)setHeight:(double)height{
_width = height;
_height = height;
}
@end
实践了里氏替换原则:
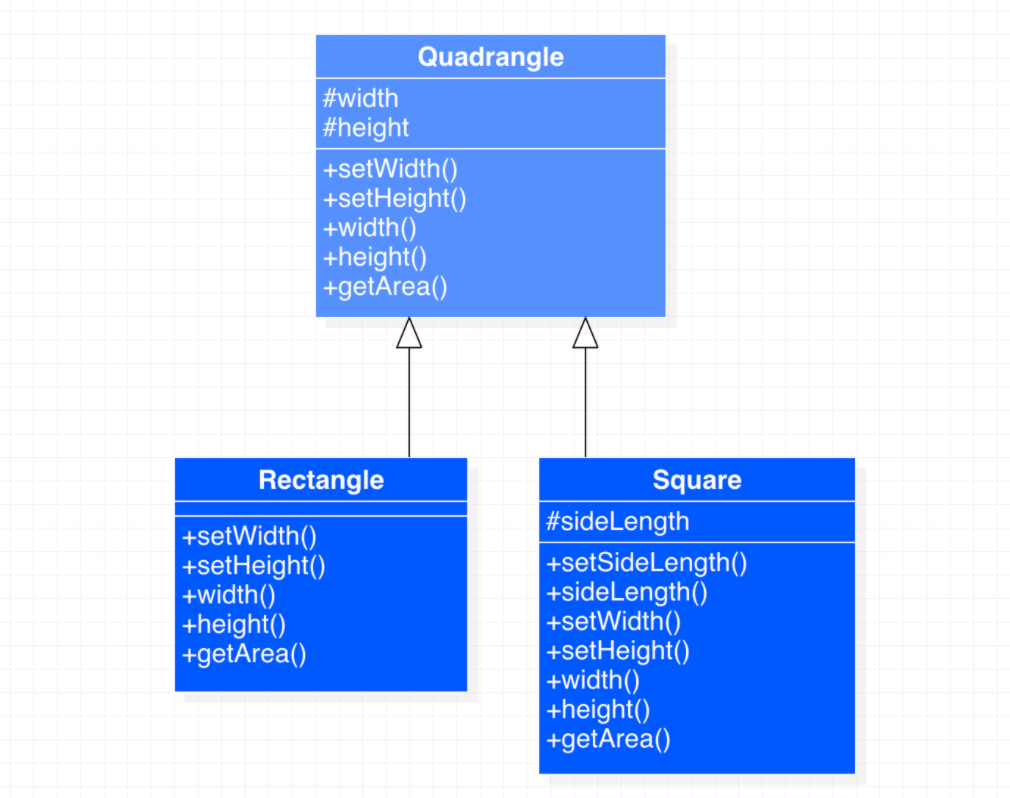
//================== Quadrangle.h ==================
@interface Quadrangle : NSObject
{
@protected double _width;
@protected double _height;
}
- (void)setWidth:(double)width;
- (void)setHeight:(double)height;
- (double)width;
- (double)height;
- (double)getArea;
@end
//================== Rectangle.h ==================
#import "Quadrangle.h"
@interface Rectangle : Quadrangle
@end
//================== Rectangle.m ==================
@implementation Rectangle
- (void)setWidth:(double)width{
_width = width;
}
- (void)setHeight:(double)height{
_height = height;
}
- (double)width{
return _width;
}
- (double)height{
return _height;
}
- (double)getArea{
return _width * _height;
}
@end
//================== Square.h ==================
@interface Square : Quadrangle
{
@protected double _sideLength;
}
-(void)setSideLength:(double)sideLength;
-(double)sideLength;
@end
//================== Square.m ==================
@implementation Square
-(void)setSideLength:(double)sideLength{
_sideLength = sideLength;
}
-(double)sideLength{
return _sideLength;
}
- (void)setWidth:(double)width{
_sideLength = width;
}
- (void)setHeight:(double)height{
_sideLength = height;
}
- (double)width{
return _sideLength;
}
- (double)height{
return _sideLength;
}
- (double)getArea{
return _sideLength * _sideLength;
}
@end
Enjoy Reading This Article?
Here are some more articles you might like to read next: