Reverse LinkedList
Reverse Linked List
Solution (Traverse the linked list, pointing the next of each node to the previous node)
- Save the next of the current node,
next = curr.next
- Point the next of the current node to the previous node,
curr.next = prev
- Move the forward pointer back to
prev = curr
- Move the current pointer back to
curr = next
Step
1.Initial list and pre node
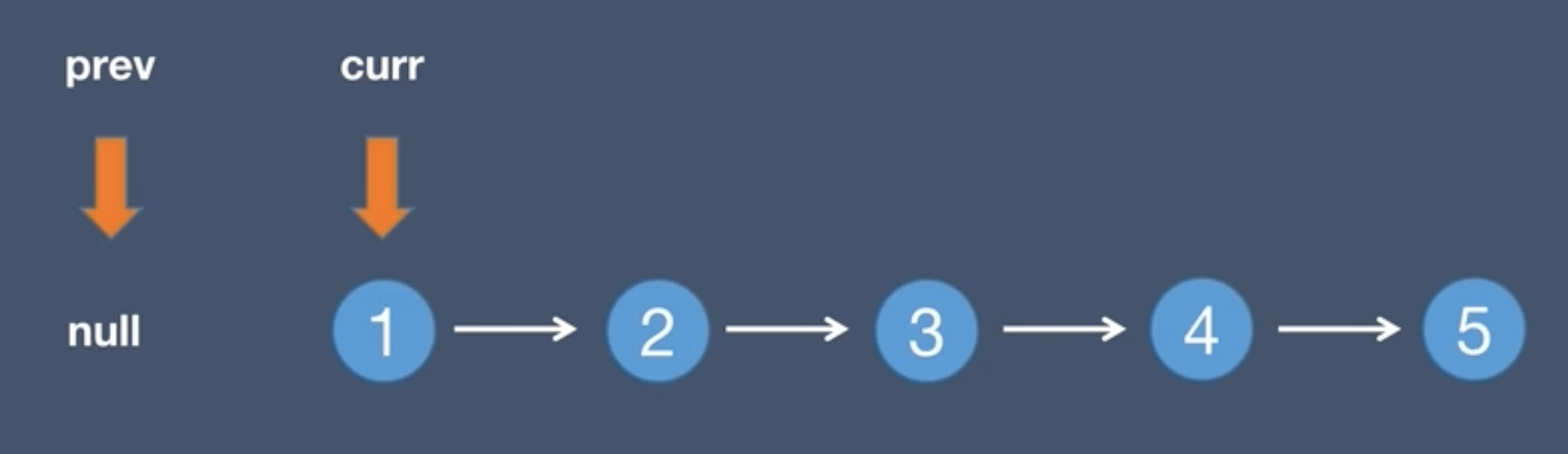
2.Initial list and next node, next = curr.next
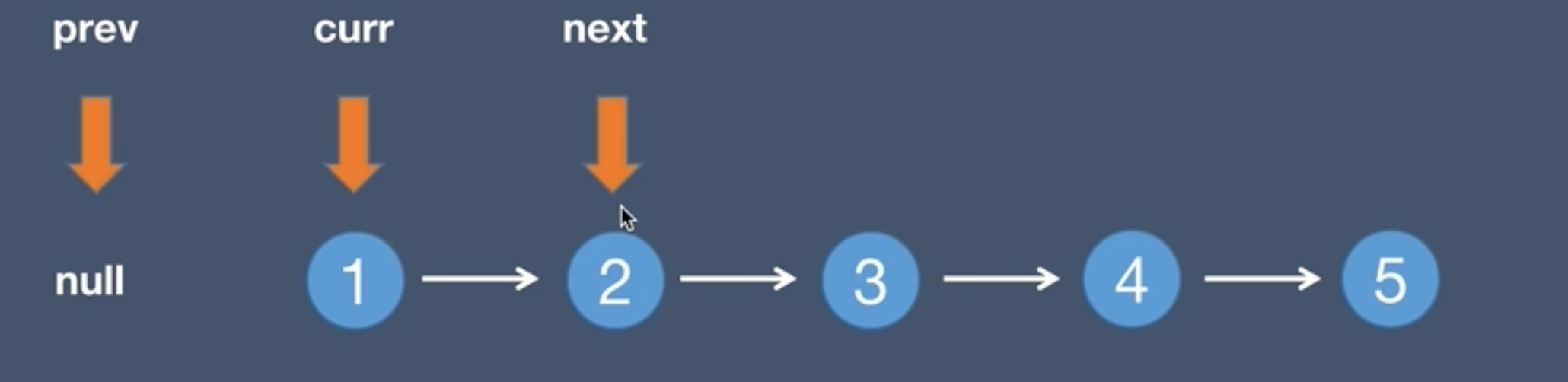
3.The current.next points to the pre node, curr.next = prev
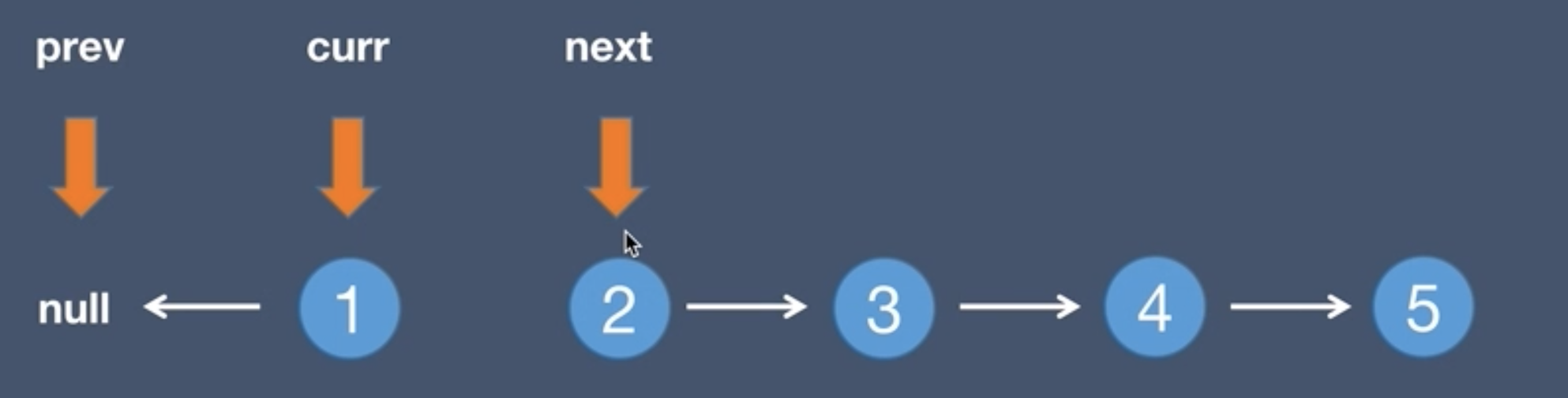
4.The pre node points to the current node, prev = curr
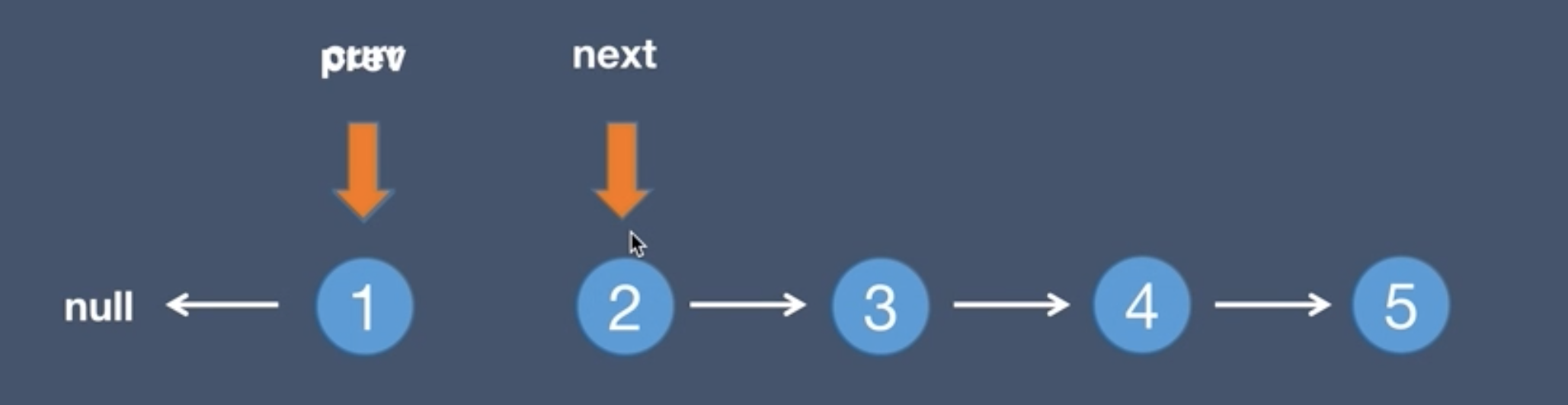
5.The current node points to the next node, curr = nex
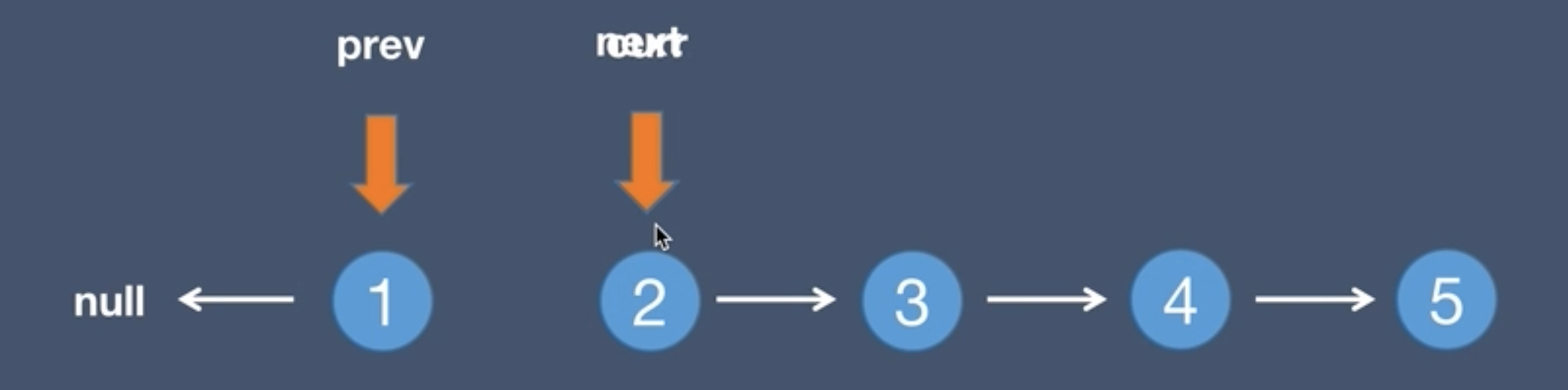
6.The next node moves to the current.next, next = curr.next
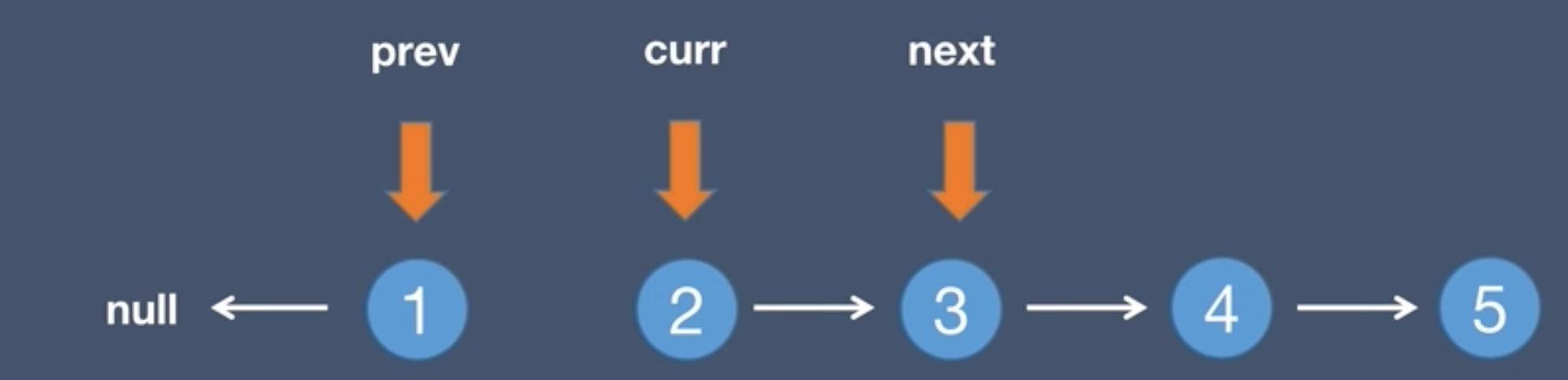
7.Loop traversal
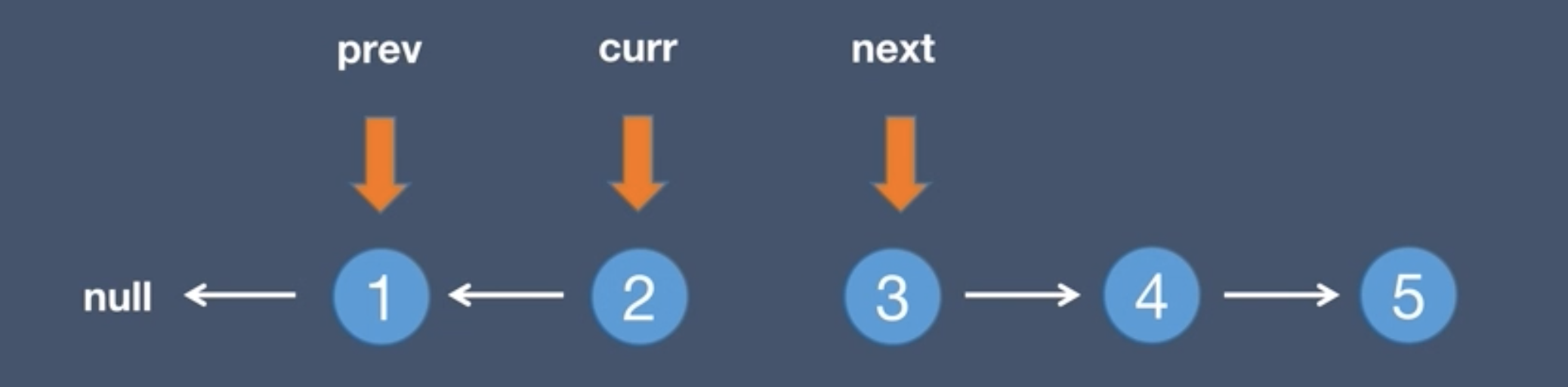
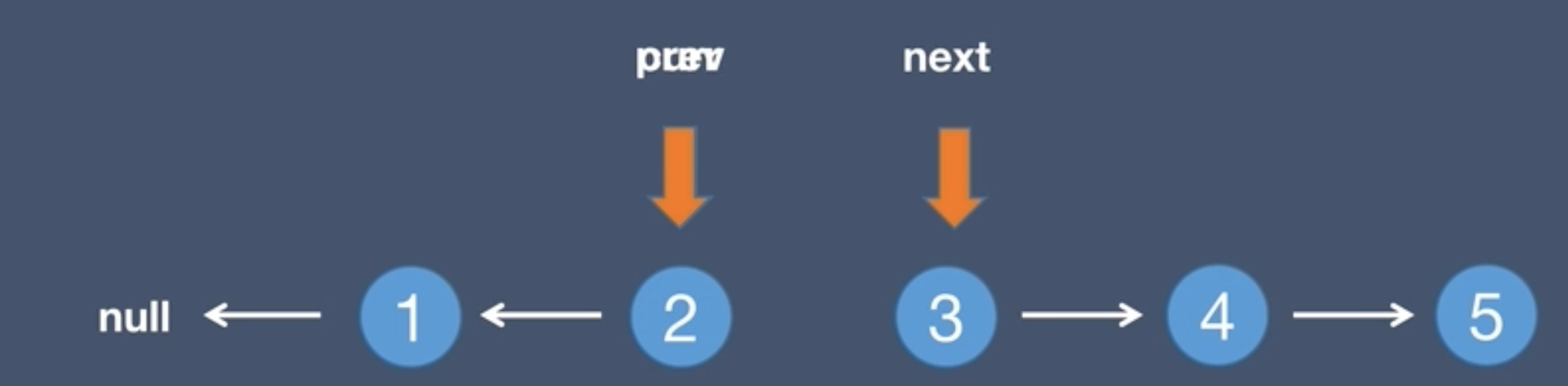
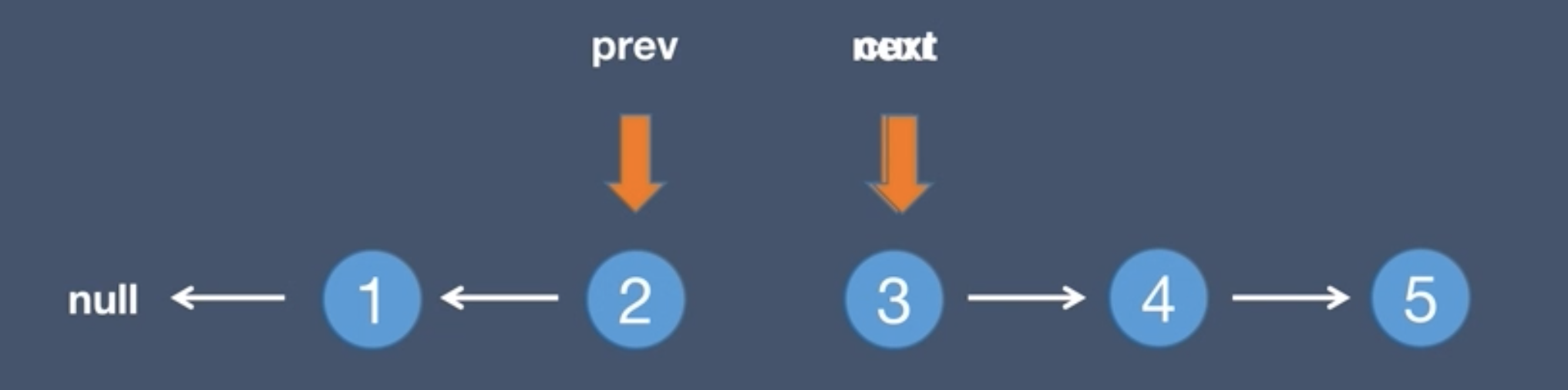
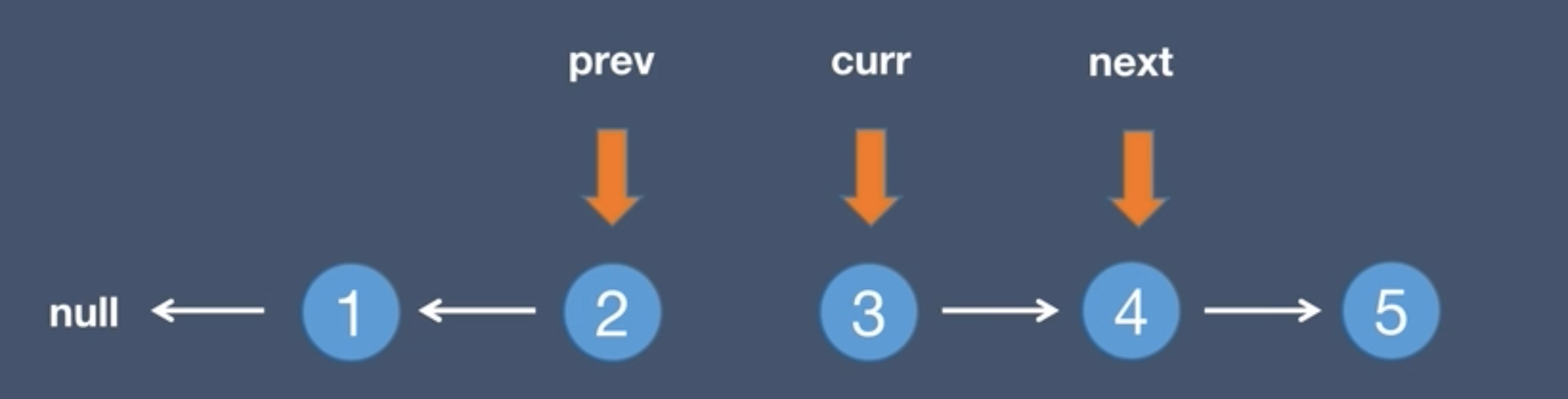
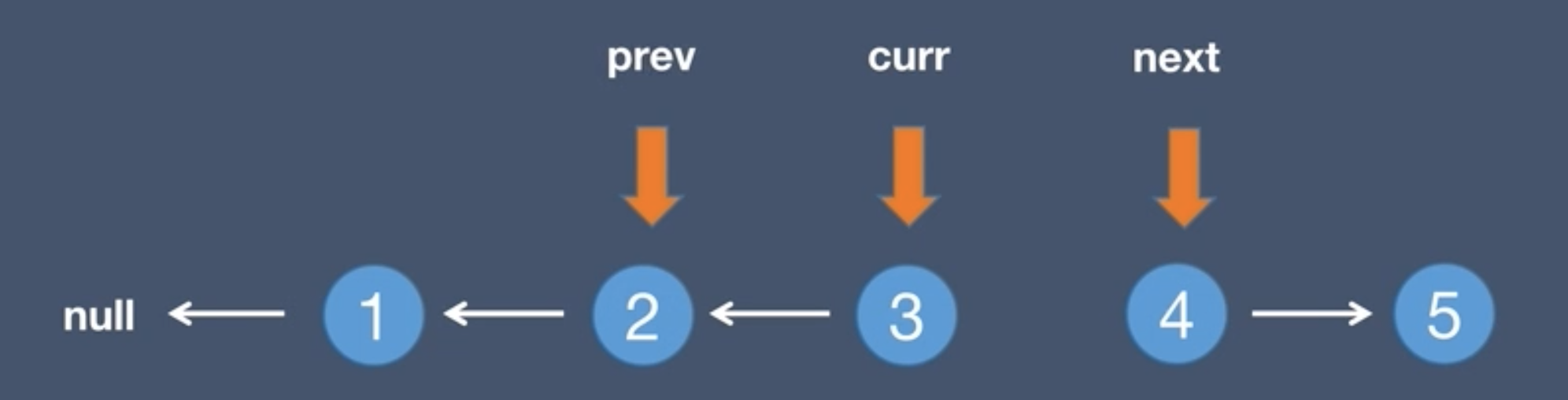
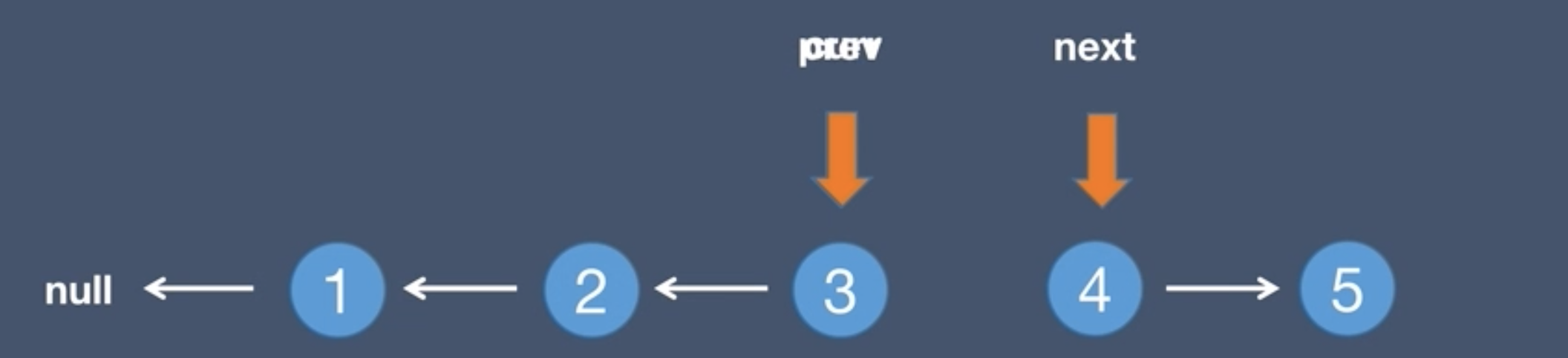
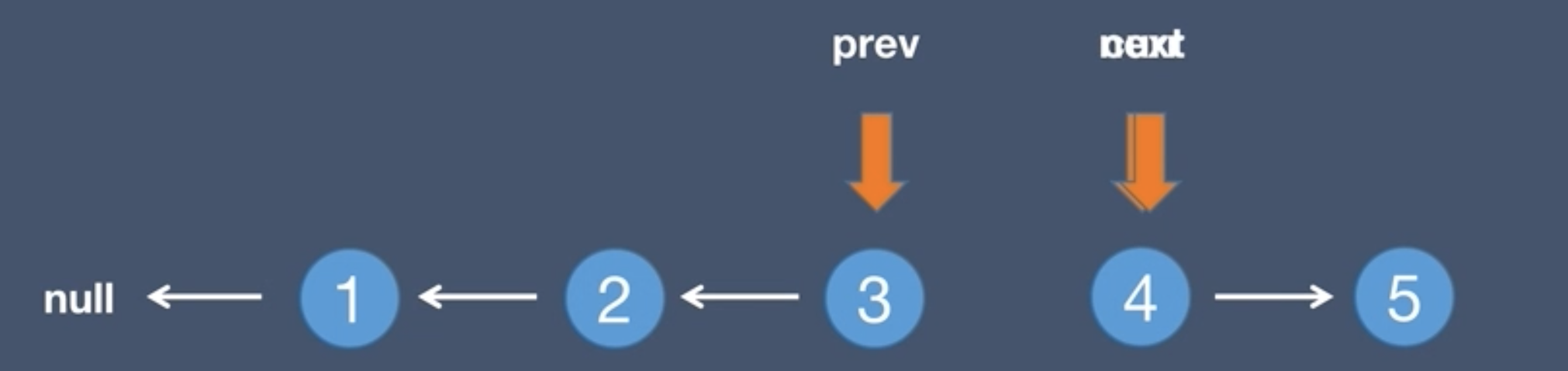
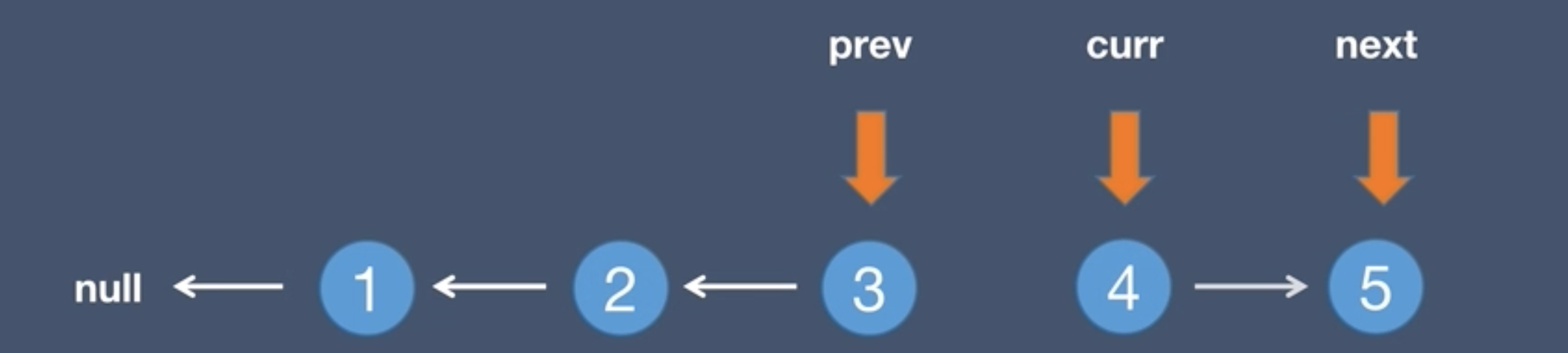
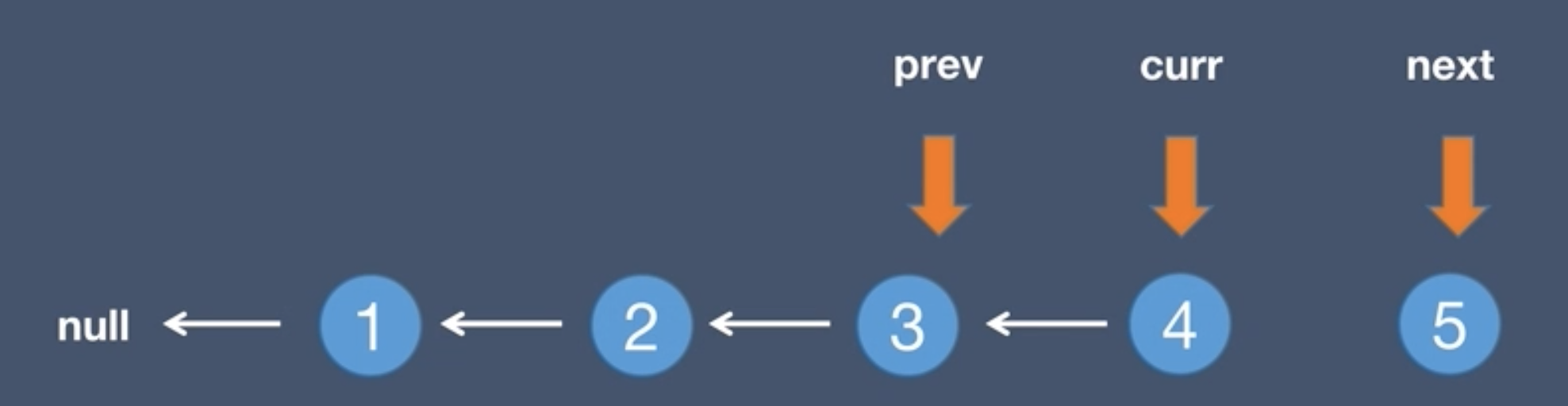
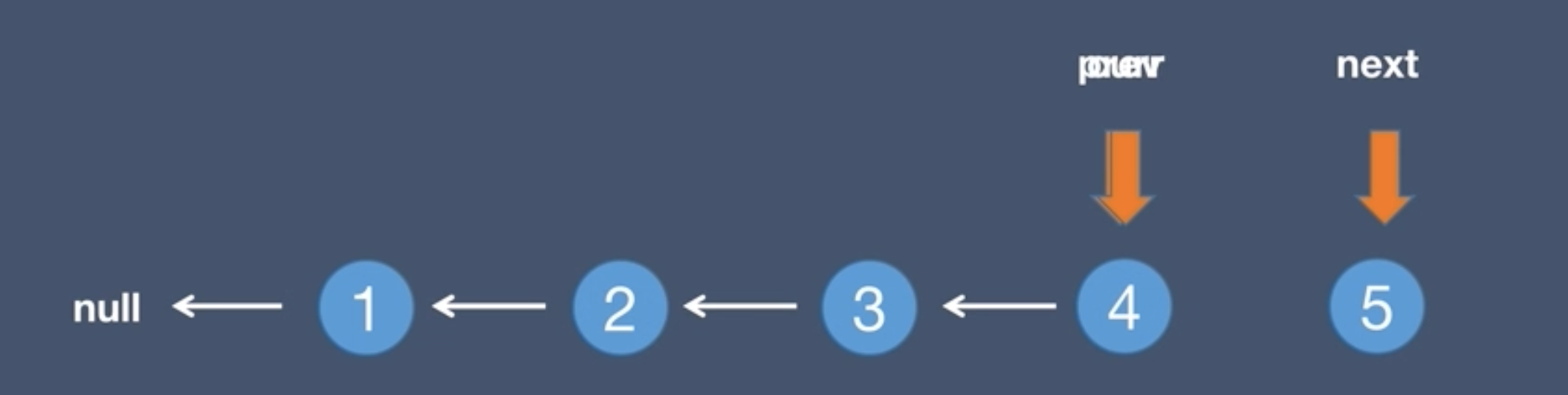
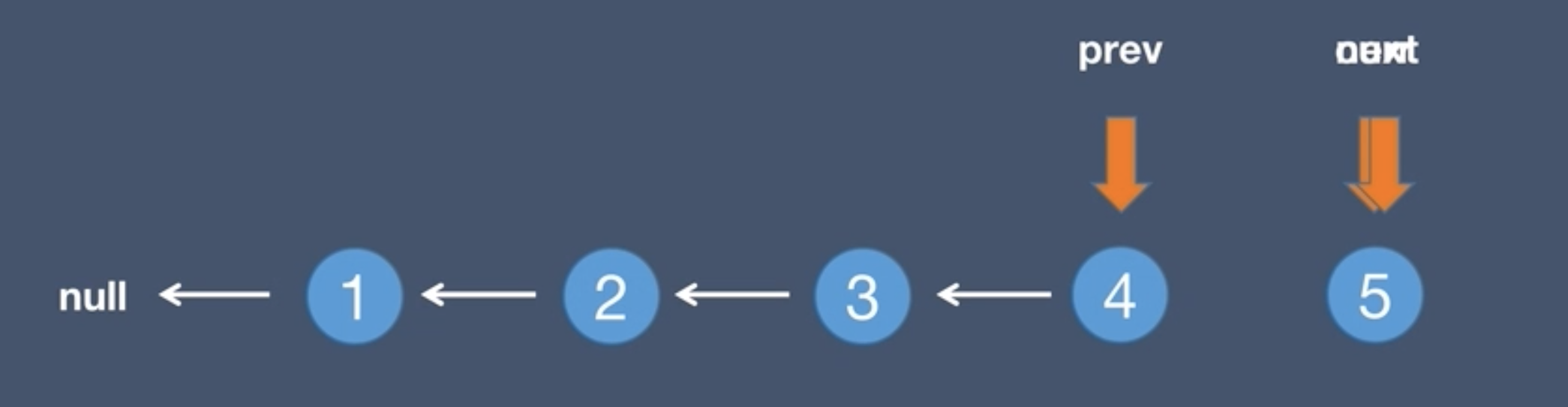
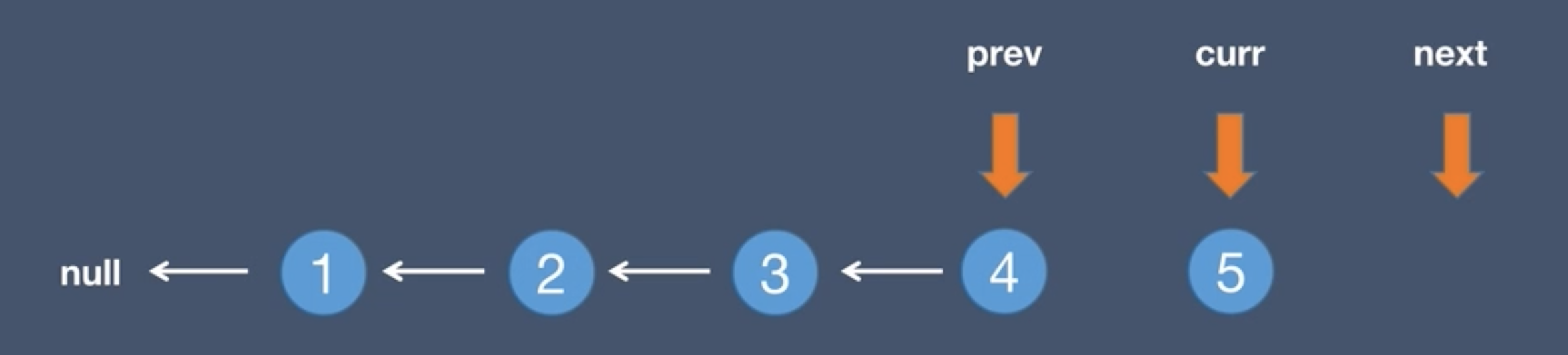
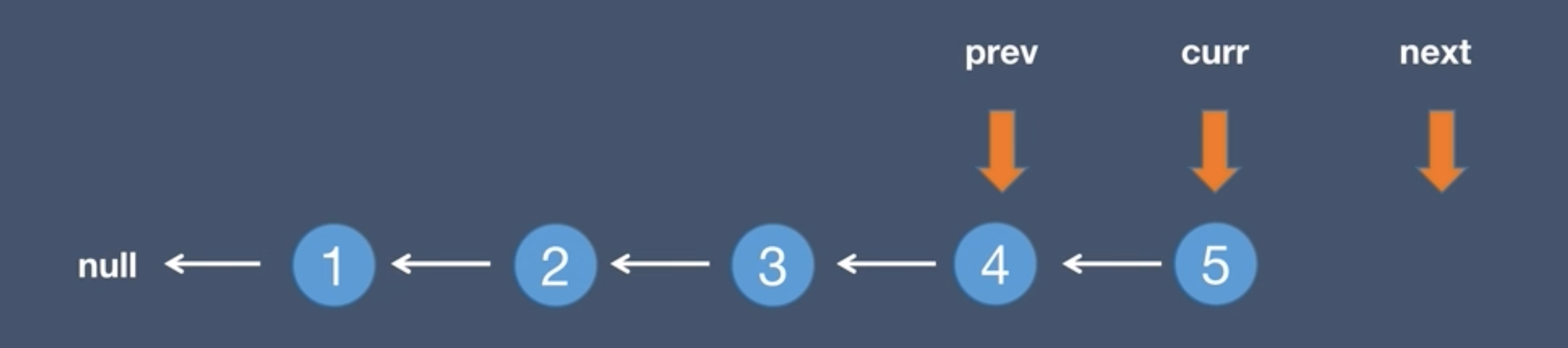
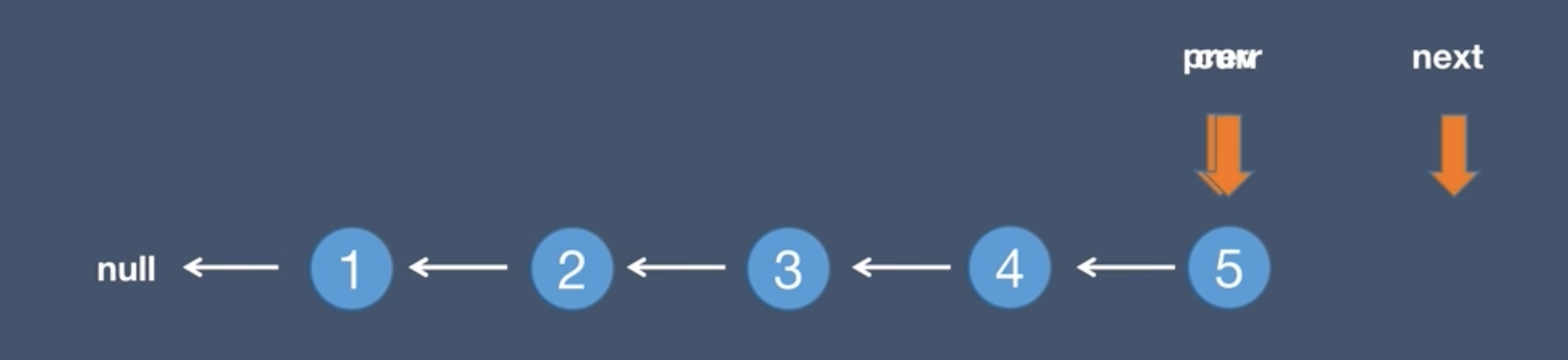
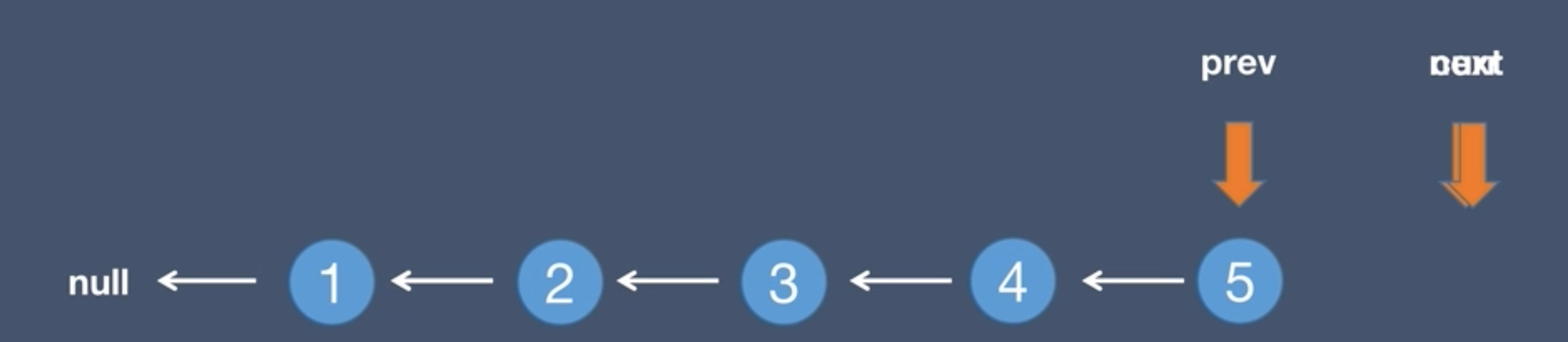
Code
package LinkedLists;
/**
* @author zhengstars
* @date 2023/08/19
*/
public class ReverseLinkedList {
public static class ListNode {
int value;
ListNode next;
public ListNode(int value) {
this.value = value;
this.next = null;
}
}
public static ListNode reverseLinkedList(ListNode head) {
ListNode prev = null;
ListNode curr = head;
ListNode next = null;
while (curr != null) {
next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
return prev;
}
}
Enjoy Reading This Article?
Here are some more articles you might like to read next: